@Chris-Schlitt Hi Chris, were you able to make it work?
I'm still trying to cross compile the package with tons of errors, so I was wondering if you had more luck..
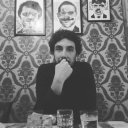
Posts made by tommaso tani
-
RE: Install/Cross Compiled Node Modules
-
Earthquake monitor with Omega2+
Since the Omega2+ offers a pretty standard GPIO interface, I decided to “go hardware” and make a small project. My idea was to make a sound every time an earthquake is reported in Italy; the all idea is pretty simple, but it can be useful to learn basic principles of IoT.
First of all, the hardware part. I’m using an active buzzer (the only one I have) that is connected to the Omega2+ and controlled by the GPIO interface through a transistor. The components I used are:
- Omega2+ with expansion board
- A breadboard
- An active buzzer
- One PNP transistor (8550)
- One Resistor (1KΩ)
- Several jumper wires
Here is the schematic for the circuit:
I connected the positive pole of the buzzer to the 5V (2nd pin of the Omega2+’s board) and the negative one to the emitter of the transistor. The collector to the ground and the base to the GPIO0 of the expansion header, through the resistor. Pretty straightforward so far. You can test from the Omega2+ web interface that, setting the GPIO0 to output, the buzzer… will buzz.
Now, it’s time to write the code: it will be a python script that fetches the QuakeML feed from the INGV website every minute and, if a new earthquake is found, makes a buzz — setting the GPIO0 to output for a certain time and then back in input. It’s not elegant at all, but again it shows some basic principles of coding with this kind of hardware. The full script is available on GitHub so here I will cover just the basic instructions.
Using the GPIO interface with python requires python of course and the onionGpio module, so:opkg update opkg install python-light pyOnionGpio
You can also choose to install the complete python package instead, it depends on the space you have available on the Omega2+. First of all, I set up the GPIO interface, using the 0 channel, via the onionGpio module:
import onionGpio #Set GPIO gpioNum = 0 gpioObj = onionGpio.OnionGpio(gpioNum)
Now I’m ready to change the channel direction everytime I have to make a sound. Next, let’s fetch the QuakeML data. This is a special XML format for earthquakes’ data. There are a couple of modules for python but since my goal is pretty simple, I will deal with it like a standard XML input. I’ll use urllib2 and xmltodict (which you need to install via pip) to parse the file and create a dictionary. I built an easy function that download the data, check the field publicID of the last record and update the id if it represents a new earthquake. In this case, I also switch the channel direction to output and then back to input, after a small amount of time (default 0.5 seconds, the buzzer is really annoying!). I use gpioObj.setOutputDirection(0) and gpioObj.setInputDirection() to command the GPIO interface. The whole function now is like this:
def checkLast(): global lastId fsock = urllib2.urlopen(requestURL) #requestURL is the QuakeML handler from the INGV file data = fsock.read() fsock.close() data = xmltodict.parse(data) last = 0 newId = data[‘q:quakeml’][‘eventParameters’][‘event’][0][‘@publicID’] if(lastId != newId): print “* Last earthquale:”,data[‘q:quakeml’][‘eventParameters’][‘event’][0][‘description’][‘text’],”{“,newId,”}” lastId = newId #Buzz it! status = gpioObj.setOutputDirection(0) time.sleep(0.5) status = gpioObj.setInputDirection() else: print “* No new earthquake, last id:”, lastId print ‘ — -’
Then, I’ll call the function every minute with an amazing infinite loop (I warned you it was not elegant at all):
while True: checkLast() time.sleep(60)
The version of the script that you can find on GitHub contains some small adjustments, like the possibility to specify a minimum magnitude and the lenght of the buzz. Since I want to lunch the script via SSH and then keep it going without keep the session open, I recommend you to use nohup to keep the script running:
nohup python earthquakes-italy.py </dev/null >quakes.log 2>&1 &
In this way, I have a python process detached from the shell that is up and running and buzzing every time a new earthquake is reported by INGV.
Ps. INGV is the Italian Institute for Geology and Volcanology and provides data related to Italian soil. You can easily adapt the script to fetch data from USGS web API which cover basically the whole world — however, I used the Italian source for my project since its data are more reliable for the country.
(See original post on Medium)
-
RE: Ruby gems that require building native extensions
@Lazar-Demin thank you! Do you know any workaround to get this to work? I really need those headers
-
RE: Ruby gems that require building native extensions
Hi! Have you eventually managed to find how to install ruby-dev? It Is really annoying being unable to build native extensions!
-
RE: Impossible to import OpenSSL module in Python
So, apparently I solved the issue with the opkg (didn't update, I downloaded the .ipk package from the repo and installed manually).
I solved the OpenSSL, now I'm struggling with another module (Twisted). LOLThank you so much anyway!
-
RE: Impossible to import OpenSSL module in Python
Moreover, I run into another funny paradox. I suspect I need to install python-pyopenssl in order to have to right module but opkg is not on my side with this:
root@Omega:/etc/opkg# opkg list | grep -i python-pyopenssl python-pyopenssl - 17.2.0-1 root@Omega:/etc/opkg# opkg info python-pyopenssl Package: python-pyopenssl Version: 17.2.0-1 Depends: libc, python-light, python-cryptography, python-six Status: install user not-installed Architecture: mipsel_24kc
So, it is listed. Good. an
opkg update
just to be sure, but then:root@Omega:/etc/opkg# opkg install python-pyopenssl Installing python-pyopenssl (17.2.0-1) to root... Collected errors: * opkg_download_pkg: Package python-pyopenssl is not available from any configured src. * opkg_install_pkg: Failed to download python-pyopenssl. Perhaps you need to run 'opkg update'? * opkg_install_cmd: Cannot install package python-pyopenssl.
-
RE: Impossible to import OpenSSL module in Python
@György-Farkas said in Impossible to import OpenSSL module in Python:
Well, the point is that I'm using the Twisted module and it has# System imports from OpenSSL import SSL supported = True
The module is installed tho:
root@Omega:~# opkg list-installed | grep python python - 2.7.13-6 python-base - 2.7.13-4 python-cffi - 1.8.3-1 python-codecs - 2.7.13-6 python-compiler - 2.7.13-4 python-crypto - 2.6.1-1 python-ctypes - 2.7.13-4 python-db - 2.7.13-4 python-decimal - 2.7.13-4 python-distutils - 2.7.13-4 python-email - 2.7.13-6 python-gdbm - 2.7.13-4 python-light - 2.7.13-4 python-logging - 2.7.13-6 python-multiprocessing - 2.7.13-4 python-ncurses - 2.7.13-4 python-openssl - 2.7.13-6 python-ply - 3.9-1 python-pycparser - 2.14-3 python-pydoc - 2.7.13-4 python-sqlite3 - 2.7.13-4 python-unittest - 2.7.13-4 python-xml - 2.7.13-4 root@Omega:~# opkg files python-openssl Package python-openssl (2.7.13-6) is installed on root and has the following files: /usr/lib/python2.7/lib-dynload/_hashlib.so /usr/lib/python2.7/lib-dynload/_ssl.so
Still haven't find a way to fix it, damn.
-
Impossible to import OpenSSL module in Python
Hi everyone,
I'm kind of going mad on this.
I have a simple script that imports ssl from OpenSSL module:from OpenSSL import ssl
of course I installed the library via OPKG and made any kind of test and manual install you can imagine but
ImportError: No module named OpenSSL
There's no way I can load it. I'm going crazy.
Thank you guys - I hope I'm missing something really basic. -
RE: Demo Wifi AP sniffer
@Hank-Hill said in Demo Wifi AP sniffer:
Ekhau
As far I as know, there have been many problems (read: impossible) to set the Omega in monitor mode - see this post.
I didn't try yet but I think that with the GPS expansion it could be possible releasing something like that.. -
Demo Wifi AP sniffer
Since it offers a really easy (I mean, really really easy) way to configure it as wifi access point, my first project was try to run a traffic sniffer / inspector — technically, I need to use it to demostrate how using free wifi is insecure (MITM kind attacks). Basically, we will run tcpdump on the Omega2+ and read the captured traffic remotely with Wireshark.
The Omega2+ runs a particular branch of OpenWRT called LEDE. This can make things quite complicated sometimes but not this time.
First, plug-in the Omega2+ and do all those sweet steps that the setup prescribes. Once done, activate the AP functionality from the web interface. Just one click.Now, every computer connected to the Omega2+ wifi will be able to use the Internet and all traffic will be bridged between the two virtual interfaces of the Omega2+. It smells MITM everywhere. So, let’s move on.
In order to install some software to sniff packets, we should re-add the official LEDE repositories: by default, just Onion packages would be active. So:vi /etc/opkg/distfeeds.conf
In the editor, re-enable the LEDE repos and comment out the Onion ones so that you end up with i file like this:
src/gz reboot_core http://downloads.lede-project.org/snapshots/targets/ramips/mt7688/packages
src/gz reboot_base http://downloads.lede-project.org/snapshots/packages/mipsel_24kc/base
#src/gz reboot_onion http://repo.onion.io/omega2/packagessrc/gz reboot_luci http://downloads.lede-project.org/snapshots/packages/mipsel_24kc/luci
src/gz reboot_packages http://downloads.lede-project.org/snapshots/packages/mipsel_24kc/packages
src/gz reboot_routing http://downloads.lede-project.org/snapshots/packages/mipsel_24kc/routing
src/gz reboot_telephony http://downloads.lede-project.org/snapshots/packages/mipsel_24kc/telephony
#src/gz omega2_core http://repo.onion.io/omega2/packages/core
#src/gz omega2_base http://repo.onion.io/omega2/packages/base
#src/gz omega2_packages http://repo.onion.io/omega2/packages/packages
src/gz omega2_onion http://repo.onion.io/omega2/packages/onionReboot the Omega2+ and update package list:
opkg update
If you get an error about the verification of the signature, you need to disable it (this is an issue just because the firmware is compiled by Onion so this verification is not going well). Open the opkg configuration file:
vi /etc/opkg.conf
and comment out the line of the verification of the signature. Try again updating the package list, it should work. We can now install tcpdump, that is the software we are going to use to sniff the traffic (but you already know that). Easy as 1 2 3:
opkg install tcpdump
Incredibly, it’s done. Nothing more needed, YAY!
Now, we’re going to create a pipe on our OSX/Linux system that will receive the data capture via an SSH connection from the Omega2+ (supposed that we still are in the same wifi network) and open Wireshark. On our pc:mkfifo /tmp/remote
wireshark -k -i /tmp/remoteThis will create the pipe and open a Wireshark instance with the pipe as input. Then, in an other terminal window we’ll start tcpdump via ssh:
ssh root@192.168.3.1 "tcpdump -s 0 -U -n -w - -i br-wlan not port 22" > /tmp/remote
Of course, if you changed Omega2+ configuration you will need to change IP address. We also added “not port 22” filter to take out our ssh connection out of the capture: you can add here whatever filter you want.
Immediately, you will see the Wireshark window populated with all the traffic going through the Omega2+.Unfortunately, the type of distro the runs on Omega2+ doesn’t allow to run all that out-of-the-box software to carry a proper and effective MITM attack: anyway, the purpose of my project was to demonstrate to an audience that with a small device powered with a battery pack (so 100% portable) can be used in a public place to steal any kind of data. Another option is to expand the internal memory of the Omega2+ and save the traffic dump in a file that could be later analyzed with Wireshark.